FREE Code Snippet – Restrict Access To Media Files
Managing a WordPress website with multiple users can sometimes be challenging, especially regarding media uploads.
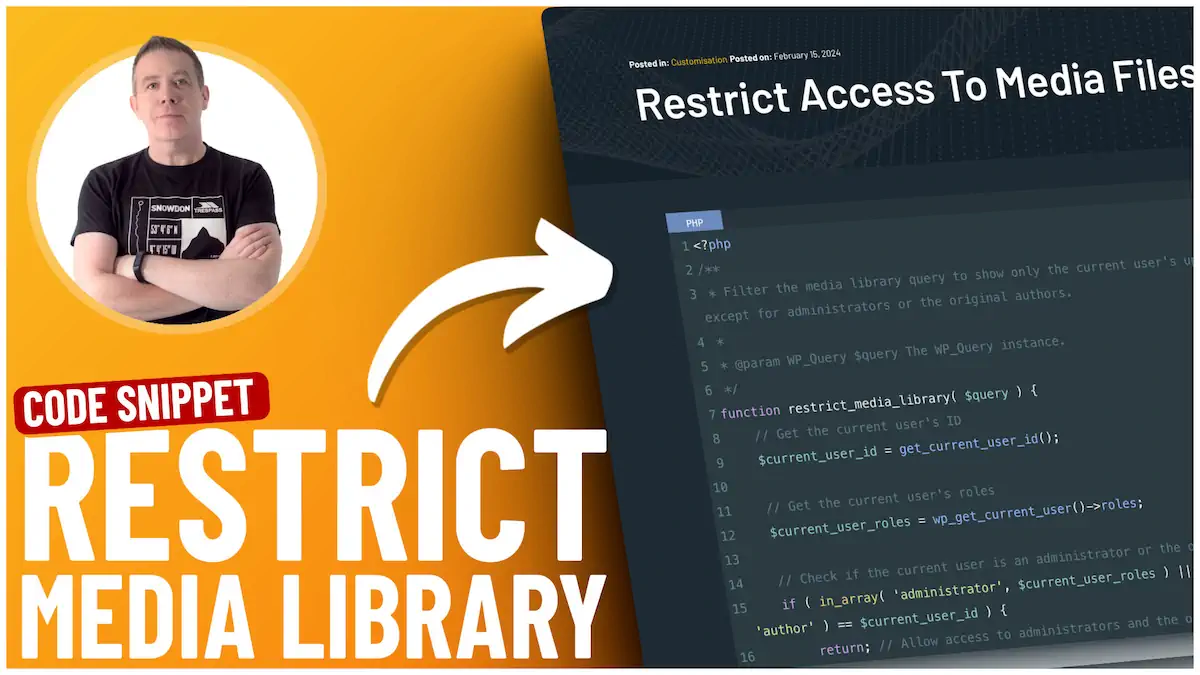
You may want your editors and authors to upload media to the site, but you might also wish to ensure they can only see and manage their own uploads. This can help maintain privacy and organization, particularly on websites with many users contributing content.
Fortunately, you don’t necessarily need a plugin to achieve this functionality. With a simple code snippet, you can restrict users to only seeing their own media files in the WordPress admin media library. Here’s how you can do it:
Step 1: Understanding the Requirement
As an administrator of a WordPress site, you have access to all media files uploaded by any user. However, for other roles like editors or authors, you may want to limit their access to only the media they upload. This ensures that users are not overwhelmed with content and have a clear view of their work.
Step 2: Using a Code Snippet
Instead of using a plugin, you can insert a custom PHP code snippet into your site to restrict media library access. You can add this code to your theme’s `functions.php` file, use a child theme to prevent losing changes on theme updates or use a plugin like Fluent Snippets to manage your code snippets.
Step 3: The Code Explained
The code snippet you’ll use checks the current user’s role and modifies the media query accordingly. If the user is an administrator, they will see all files. If the user has any other role, they will only see the files they uploaded. The code does this by:
- Creating a custom function to restrict media library access.
- Checking the logged-in user’s ID.
- Determining the user’s role (administrator, editor, author, etc.).
- Modifying the media library query to filter results based on the user’s role and ID.
Step 4: Implementing the Code
Once you have the code snippet, you can add it to your site using your preferred method. If you’re using a code snippet plugin, you would typically paste the code into a new snippet field, give it a name, and activate it. If you’re adding it directly to your `functions.php` file, you would paste it at the end of the file.
<?php
/**
* Filter the media library query to show only the current user's
uploads, except for administrators or the original authors.
*
* @param WP_Query $query The WP_Query instance.
*/
function restrict_media_library( $query ) {
// Get the current user's ID
$current_user_id = get_current_user_id();
// Get the current user's roles
$current_user_roles = wp_get_current_user()->roles;
// Check if the current user is an administrator or the original author
if ( in_array( 'administrator', $current_user_roles ) || $query->get( 'author' ) == $current_user_id ) {
return; // Allow access to administrators and the original authors
}
// Retrieve the original author's ID from the queried post
$original_author_id = (int) $query->get( 'author' );
// Check if the current user is the original author
if ( $original_author_id === $current_user_id ) {
return; // Allow access to the original author
}
// Set the author query parameter to the current user's ID
$query->set( 'author', $current_user_id );
}
add_action( 'pre_get_posts', 'restrict_media_library' );
Step 5: Testing the Functionality
After implementing the code, it’s important to test it. Log in as an administrator to ensure you can still see all media files. Then, log in as another user role, such as an editor or author, and check the media library. Users with these roles should now only see their uploads.
Conclusion
By following these steps, you can easily restrict users to only seeing their own media files in the WordPress admin without needing additional plugins. This helps with site organization and enhances privacy and efficiency for your users. Remember to always back up your site before making changes to the code, and if you’re not comfortable editing PHP code, consider reaching out to a professional.
Community
Join the WPTuts Academy Today
Join over 400 members and gain access to a vibrant community of web designers today.
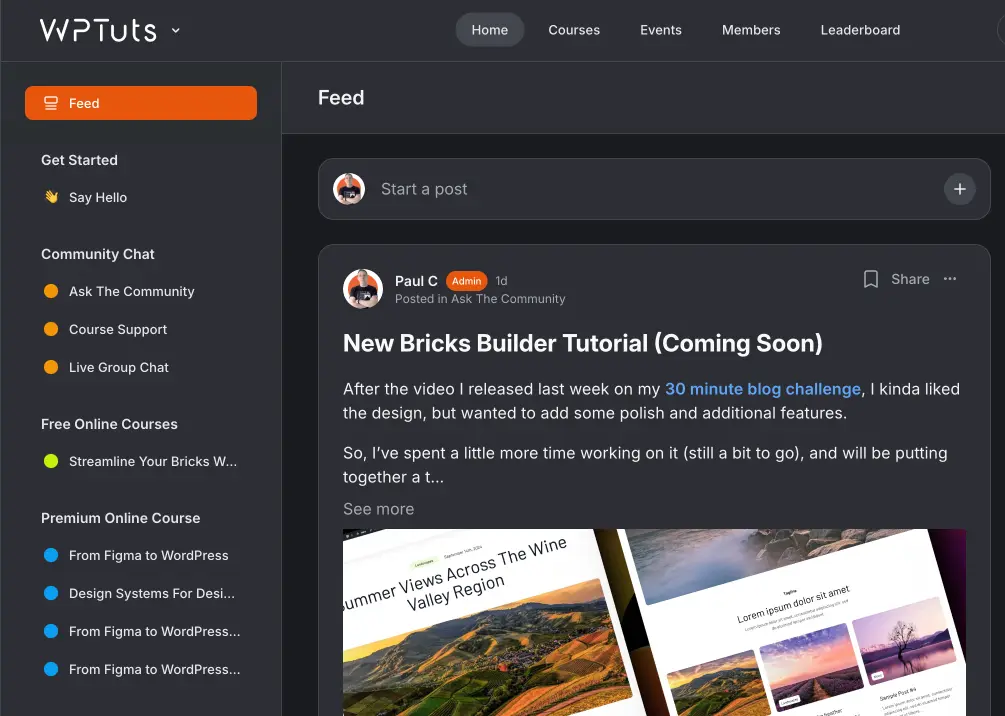